How To Integrate Google Places Autocomplete in Angular 2022
Google Place Library enables your application to search for places(geographic locations). In this article, we will integrate google place autocomplete in the angular project.
1. Let's install @angular/cli package.
npm i -g @angular/cli
2. Create a new angular project.
ng new angular-google-places-autocomplete
3. Install ngx-google-places-autocomplete package.
npm i ngx-google-places-autocomplete
4. Import GooglePlaceModule in your module.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { GooglePlaceModule } from 'ngx-google-places-autocomplete';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
GooglePlaceModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
5. Open angular.json and add ngx-google-places-autocomplete inside allowedCommonJsDependencies
"architect": {
"build": {
"builder": "@angular-devkit/build-angular:browser",
"options": {
"allowedCommonJsDependencies": [
"ngx-google-places-autocomplete"
],
"outputPath": "dist/angular-google-places-autocomplete",
"index": "src/index.html",
6. Install @types/googlemaps package.
npm i --save-dev @types/googlemaps
7. Open tsconfig.app.json and update "types" property to ["googlemaps"] inside compilerOptions.
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/app",
"types": ["googlemaps"]
},
"files": [
"src/main.ts",
"src/polyfills.ts"
],
"include": [
"src/**/*.d.ts"
]
}
8. Next, we need to add Google Map javascript API. You can do this by three ways
(a). Script Tag
(a1). src/index.html
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>AngularGooglePlacesAutocomplete</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<app-root></app-root>
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&libraries=places&language=en"></script>
</body>
</html>
In this way, the google library is ready on app load in the browser.
(a2). src/app/app.component.html
<div class="row mt-3">
<div class="col-6 offset-3">
<input class="form-control"
ngx-google-places-autocomplete
[options]="options"
(onAddressChange)="handleAddressChange($event)"
/>
</div>
</div>
(a3). src/app/app.component.ts
import { Component } from '@angular/core';
import { Address } from 'ngx-google-places-autocomplete/objects/address';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
options: any = {
componentRestrictions: { country: 'IN' }
}
handleAddressChange(address: Address) {
console.log(address.formatted_address)
console.log(address.geometry.location.lat())
console.log(address.geometry.location.lng())
}
}
(a4). start your application and test it in the browser.
ng serve --o
(b). Angular Google Maps (AGM)
(b1). Install @agm/core package
npm install @agm/core@1.1.0
Note:- Latest version of @agm/core has a few bugs also it's in the beta state. so install version 1.1.0.
If you got "Generic type ‘ModuleWithProviders‘ requires 1 type argument(s)” error. then add "skipLibCheck": true inside compilerOptions.
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/app",
"types": ["googlemaps"],
"skipLibCheck": true
},
"files": [
"src/main.ts",
"src/polyfills.ts"
],
"include": [
"src/**/*.d.ts"
]
}
(b2). Import AgmCoreModule in your module.
import { AgmCoreModule } from '@agm/core';
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { GooglePlaceModule } from 'ngx-google-places-autocomplete';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AgmCoreModule.forRoot({
apiKey: 'YOUR_API_KEY',
libraries: ['places']
}),
GooglePlaceModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
(b3). src/app/app.component.html
<div class="row mt-3">
<div class="col-6 offset-3" *ngIf="isApiLoaded">
<input class="form-control"
ngx-google-places-autocomplete
[options]="options"
(onAddressChange)="handleAddressChange($event)"
/>
</div>
</div>
(b4). src/app/app.component.ts
import { MapsAPILoader } from '@agm/core';
import { Component, OnInit } from '@angular/core';
import { Address } from 'ngx-google-places-autocomplete/objects/address';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
isApiLoaded = false;
options: any = {
componentRestrictions: { country: 'IN' }
}
constructor(
private mapsAPILoader: MapsAPILoader
) { }
ngOnInit() {
this.mapsAPILoader.load().then(() =>{
this.isApiLoaded = true
})
}
handleAddressChange(address: Address) {
console.log(address.formatted_address)
console.log(address.geometry.location.lat())
console.log(address.geometry.location.lng())
}
}
(b5). start your application and test it in the browser.
ng serve --o
(c). Custom Script Loader - Manually Inject Script.
(c1). src/app/app.component.html
<div class="row mt-3">
<div class="col-6 offset-3" *ngIf="isApiLoaded">
<input class="form-control"
ngx-google-places-autocomplete
[options]="options"
(onAddressChange)="handleAddressChange($event)"
/>
</div>
</div>
(c2). src/app/app.component.ts
import { DOCUMENT } from '@angular/common';
import { Component, ElementRef, Inject, OnInit } from '@angular/core';
import { Address } from 'ngx-google-places-autocomplete/objects/address';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
isApiLoaded = false;
options: any = {
componentRestrictions: { country: 'IN' }
}
constructor(
@Inject(DOCUMENT) private document: Document,
private elementRef: ElementRef
) { }
ngOnInit() {
this.loadScript().then(() =>{
this.isApiLoaded = true
})
}
loadScript() {
return new Promise((resolve, reject) => {
const element = this.document.createElement('script');
element.type = 'text/javascript';
element.src = 'https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&libraries=places&language=en';
element.onload = resolve;
element.onerror = reject;
this.elementRef.nativeElement.appendChild(element);
})
}
handleAddressChange(address: Address) {
console.log(address.formatted_address)
console.log(address.geometry.location.lat())
console.log(address.geometry.location.lng())
}
}
(c3). start your application and test it in the browser.
ng serve --o
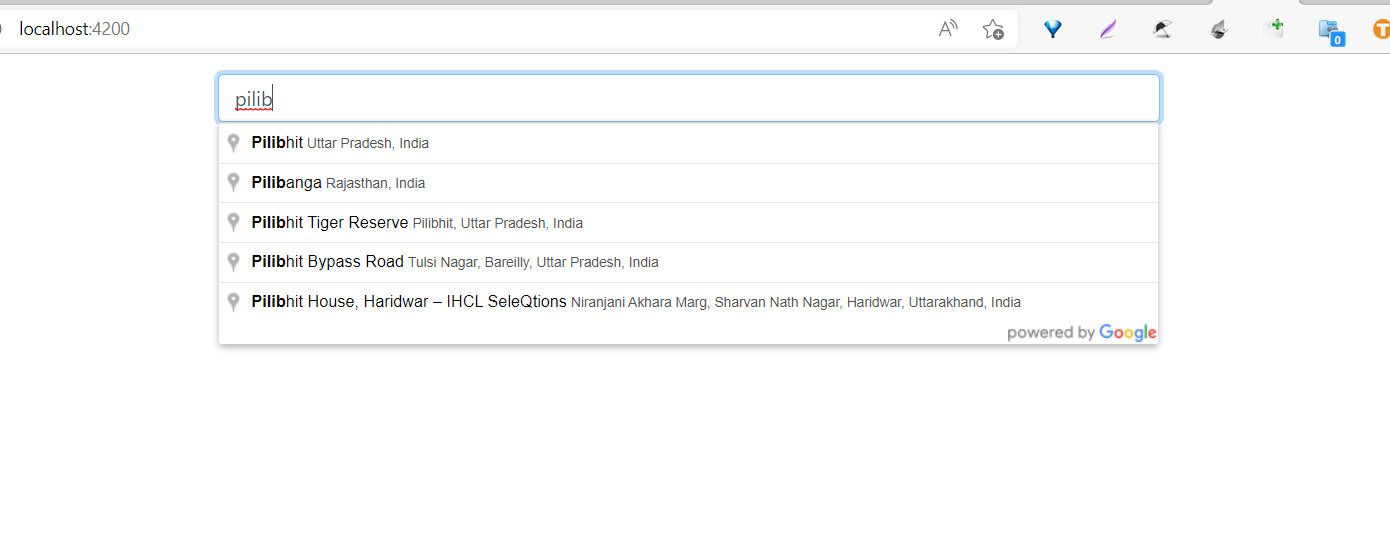
Extras:- Place API Options
1. You can pass multiple countries in options.
options: any = {
componentRestrictions: { country: ['IN', 'US'] }
}
2. You can also pass place types in options.
options: any = {
types: ['hospital', 'pharmacy', 'bakery', 'country'],
componentRestrictions: { country: 'IN' }
}
Ref: Checkout https://developers.google.com/maps/documentation/javascript/supported_types
3. If you need to use place autocomplete inside a modal(popup). then you need to increase the z-index of .pac-container
style.css
.pac-container{
z-index:9999 !important;
}
Checkout my full angular-google-places-autocomplete example.
https://github.com/ultimateakash/angular-google-places-autocomplete
If you facing any issues. don't hesitate to comment below. I will be happy to help you.
Thanks.
How To Integrate Google Maps in Angular with Angular Google Maps 2022
Leave Your Comment