How To Integrate Google Maps in Angular with Angular Google Maps 2022
Angular Google Maps (AGM) provides a set of functions for implementing Google Maps API. In this tutorial, we will integrate the google map in the angular project step by step.
1. Let's install @angular/cli package.
npm install -g @angular/cli
2. Create a new angular project.
ng new angular-google-maps
3. Install @agm/core package inside the project.
npm install @agm/core@1.1.0
Note:- Latest version of @agm/core has a few bugs also it's in the beta state. so install version 1.1.0.
4. Install @types/googlemaps package.
npm install --save-dev @types/googlemaps
5. Open tsconfig.app.json and update "types" property to ["googlemaps"] inside compilerOptions.
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/app",
"types": ["googlemaps"]
},
"files": [
"src/main.ts",
"src/polyfills.ts"
],
"include": [
"src/**/*.d.ts"
]
}
Note:- If you got "Generic type ‘ModuleWithProviders‘ requires 1 type argument(s)” error. then add "skipLibCheck": true inside compilerOptions.
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/app",
"types": ["googlemaps"],
"skipLibCheck": true
},
"files": [
"src/main.ts",
"src/polyfills.ts"
],
"include": [
"src/**/*.d.ts"
]
}
6. Open app.module.ts and import AgmCoreModule
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AgmCoreModule } from '@agm/core';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AgmCoreModule.forRoot({
apiKey: 'Your Google API Key'
})
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Note:- Don't forget to update your Google API Key.
7. Open styles.css file and add this css.
agm-map {
height: 400px;
}
Note:- It is really important that you define the height of agm-map. Otherwise, you won't see a map on the page.
8. Open app.component.ts and add this code.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
zoom: number = 15;
lat: number = 28.626137;
lng: number = 79.821603;
lastInfoWindow: any;
markers: any[] = [
{
lat: 28.625485,
lng: 79.821091,
label: { color: 'white', text: 'P1' },
draggable: true
},
{
lat: 28.625293,
lng: 79.817926,
label: { color: 'white', text: 'P2' },
draggable: false
},
{
lat: 28.625182,
lng: 79.814640,
label: { color: 'white', text: 'P3' },
draggable: true
}
]
markerClicked(marker: any, index: number, infoWindowRef: any) {
if (this.lastInfoWindow) {
this.lastInfoWindow.close();
}
this.lastInfoWindow = infoWindowRef;
console.log(marker, index);
}
mapClicked($event: any) {
this.markers.push({
lat: $event.coords.lat,
lng: $event.coords.lng,
draggable: true
});
}
markerDragEnd($event: any, index: number) {
console.log($event.coords.lat);
console.log($event.coords.lng);
}
}
9. Open app.component.html and add this code.
<h1 class="text-center">Angular Google Maps</h1>
<agm-map
[latitude]="lat"
[longitude]="lng"
[zoom]="zoom"
[disableDefaultUI]="false"
[zoomControl]="false"
(mapClick)="mapClicked($event)">
<agm-marker
*ngFor="let marker of markers; let i = index"
(markerClick)="markerClicked(marker, i, infoWindowRef)"
[latitude]="marker.lat"
[longitude]="marker.lng"
[label]="marker.label"
[markerDraggable]="marker.draggable"
(dragEnd)="markerDragEnd($event, i)">
<agm-info-window #infoWindowRef>
<b>{{marker.lat}}, {{marker.lng}}</b>
</agm-info-window>
</agm-marker>
</agm-map>
Note:- you can easily add/remove markers. also, you can pass additional properties in the marker object based on your requirement.
(a). Add a marker
this.markers.push({
lat: 28.625043,
lng: 79.810135,
label: { color: 'white', text: 'P4' },
draggable: true
})
(b). Remove a marker
Example:- Remove P4 marker
this.markers = this.markers.filter((marker: any) => marker.label.text != 'P4');
(c). Update marker properties
Example:- Update P4 marker
const index = this.markers.findIndex((marker: any) => marker.label.text == 'P4');
if (index != -1) {
this.markers[index]= { ...this.markers[index], lng: 28.625019, lat: 79.808296 };
}
10. Finally run the project.
ng serve --o
it will open http://localhost:4200 in the browser.
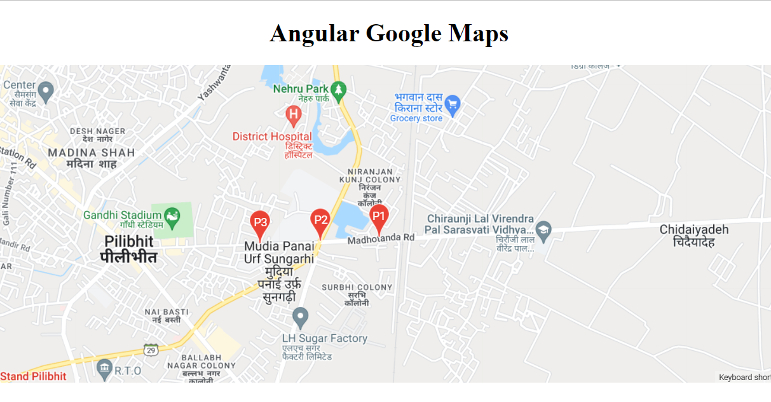
Extras:-
(a). Reverse Geocoding
getAddress(lat: number, lng: number) {
const geocoder = new google.maps.Geocoder();
const latlng = new google.maps.LatLng(lat, lng);
const request: any = {
latLng: latlng
}
return new Promise((resolve, reject) => {
geocoder.geocode(request, results => {
results.length ? resolve(results[0].formatted_address) : reject(null);
});
})
}
You can use this function by passing lat and lng.
async mapClicked($event: any) {
console.log($event.coords.lat, $event.coords.lng)
const address = await getAddress($event.coords.lat, $event.coords.lng)
console.log(address)
}
Checkout my full angular-google-maps example.
https://github.com/ultimateakash/angular-google-maps
If you facing any issues. don't hesitate to comment below. I will be happy to help you.
Thanks.
Leave Your Comment