How To Integrate Google Places Autocomplete in Laravel 2022
Google Place Library enables your application to search for places(geographic locations). In this article, we will integrate google place autocomplete in the laravel project.
1. Let's create a new laravel project.
composer create-project laravel/laravel laravel-google-places-autocomplete
2. Create a view and add the following code.
resources/views/place.blade.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="{{ asset('css/bootstrap.min.css') }}">
</head>
<body>
<div class="row mt-3">
<div class="col-6 offset-3">
<input class="form-control" placeholder="Enter Location" id="placeInput"/>
</div>
</div>
<script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&libraries=places&callback=initAutocomplete" async></script>
<script>
let autocomplete;
/* ------------------------- Initialize Autocomplete ------------------------ */
function initAutocomplete() {
const input = document.getElementById("placeInput");
const options = {
componentRestrictions: { country: "IN" }
}
autocomplete = new google.maps.places.Autocomplete(input, options);
autocomplete.addListener("place_changed", onPlaceChange)
}
/* --------------------------- Handle Place Change -------------------------- */
function onPlaceChange() {
const place = autocomplete.getPlace();
console.log(place.formatted_address)
console.log(place.geometry.location.lat())
console.log(place.geometry.location.lng())
}
</script>
</body>
</html>
Note:- Don't forget to update your Google API Key.
3. Create a controller and add the following code.
php artisan make:controller PlaceController
app/Http/Controllers/PlaceController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class PlaceController extends Controller
{
public function index()
{
return view('place');
}
}
4. Create Route
routes/web.php
<?php
use App\Http\Controllers\PlaceController;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', [PlaceController::class, 'index']);
5. Finally open http://localhost/laravel-google-places-autocomplete/public in the browser.
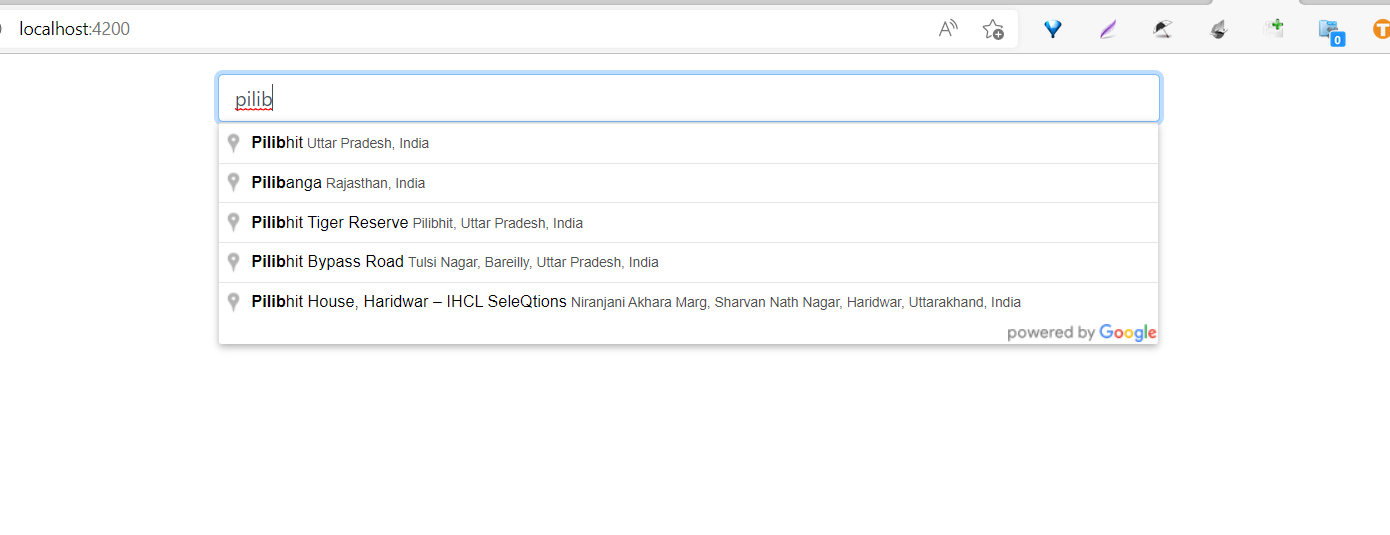
Extras:- Place API Options
1. You can pass multiple countries in options.
const options = {
componentRestrictions: { country: ['IN', 'US'] }
}
2. You can also pass place types in options.
const options = {
types: ['hospital', 'pharmacy', 'bakery', 'country'],
componentRestrictions: { country: 'IN' }
}
Ref: Checkout https://developers.google.com/maps/documentation/javascript/supported_types
3. If you need to use place autocomplete inside a modal(popup). then you need to increase the z-index of .pac-container
style.css
.pac-container{
z-index:9999 !important;
}
Checkout my full angular-google-places-autocomplete example.
https://github.com/ultimateakash/laravel-google-places-autocomplete
If you facing any issues. don't hesitate to comment below. I will be happy to help you.
Thanks.
Leave Your Comment