How To Integrate Google Places Autocomplete in React 2022
Google Place Library enables your application to search for places(geographic locations). In this article, we will integrate google place autocomplete in the react project.
1. Let's install create-react-app package.
npm i -g create-react-app
2. Create a new react project
create-react-app react-google-places-autocomplete
cd react-google-places-autocomplete
3. Install @react-google-maps/api package inside the project.
npm i @react-google-maps/api
4. Create folder components inside the src folder. Inside this folder create place.component.jsx file.
import React, { useRef } from "react";
import { StandaloneSearchBox, useJsApiLoader } from "@react-google-maps/api";
const libraries = ['places'];
const PlaceComponent = () => {
const inputRef = useRef();
const { isLoaded, loadError } = useJsApiLoader({
googleMapsApiKey: process.env.REACT_APP_GOOGLE_API_KEY,
libraries
});
const handlePlaceChanged = () => {
const [ place ] = inputRef.current.getPlaces();
if(place) {
console.log(place.formatted_address)
console.log(place.geometry.location.lat())
console.log(place.geometry.location.lng())
}
}
return (
isLoaded
&&
<StandaloneSearchBox
onLoad={ref => inputRef.current = ref}
onPlacesChanged={handlePlaceChanged}
>
<input
type="text"
className="form-control"
placeholder="Enter Location"
/>
</StandaloneSearchBox>
);
};
export default PlaceComponent;
Note:- Don't forget to update your Google API Key.
5. Open the App.js file and make the following changes.
import React from "react";
import "./App.css";
import PlaceComponent from "./components/place.component";
function App() {
return (
<div className="row mt-3">
<div className="col-6 offset-3">
<PlaceComponent />
</div>
</div>
);
}
export default App;
6. Finally start the project.
npm start
open http://localhost:3000 in the browser.
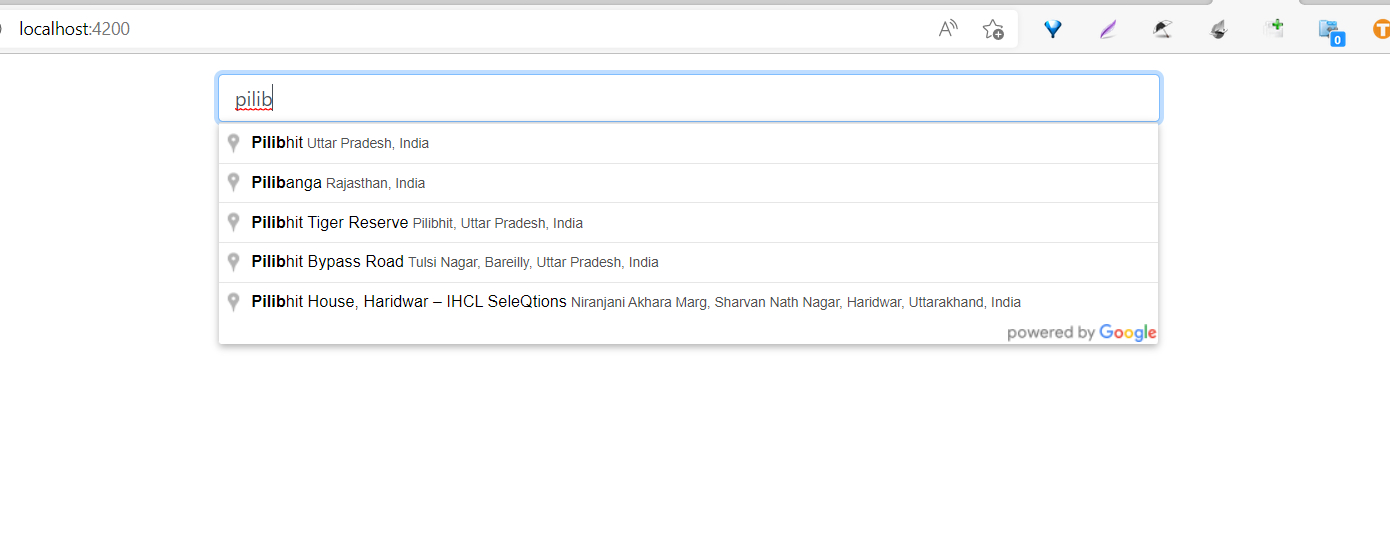
Note:- If you need to use place autocomplete inside a modal(popup). then you need to increase the z-index of .pac-container
.pac-container{
z-index:9999 !important;
}
Checkout my full react-google-places-autocomplete example.
https://github.com/ultimateakash/react-google-places-autocomplete
If you facing any issues. don't hesitate to comment below. I will be happy to help you.
Thanks.
Leave Your Comment