How To Integrate Google Maps in React 2023
@react-google-maps/api provides a set of functions for implementing Google Maps API. In this tutorial, we will integrate google maps into the react project step by step.
1. Let's install create-react-app package.
npm i -g create-react-app
2. Create a new react project
create-react-app react-google-maps
cd react-google-maps
3. Install @react-google-maps/api package inside the project.
npm i @react-google-maps/api
4. Create folder components inside the src folder. Inside this folder create map.component.jsx file.
import React, { useState } from "react";
import { GoogleMap, InfoWindow, Marker, useJsApiLoader } from "@react-google-maps/api";
const MapComponent = () => {
const { isLoaded, loadError } = useJsApiLoader({
googleMapsApiKey: process.env.REACT_APP_GOOGLE_API_KEY
});
const initialMarkers = [
{
position: {
lat: 28.625485,
lng: 79.821091
},
label: { color: "white", text: "P1" },
draggable: true
},
{
position: {
lat: 28.625293,
lng: 79.817926
},
label: { color: "white", text: "P2" },
draggable: false
},
{
position: {
lat: 28.625182,
lng: 79.81464
},
label: { color: "white", text: "P3" },
draggable: true
},
];
const [activeInfoWindow, setActiveInfoWindow] = useState(null);
const [markers, setMarkers] = useState(initialMarkers);
const containerStyle = {
width: "100%",
height: "400px",
}
const center = {
lat: 28.626137,
lng: 79.821603,
}
const mapClicked = (event) => {
console.log(event.latLng.lat(), event.latLng.lng())
}
const markerClicked = (marker, index) => {
setActiveInfoWindow(index)
console.log(marker, index)
}
const markerDragEnd = (event, index) => {
console.log(event.latLng.lat(), event.latLng.lng())
}
return (
isLoaded
&&
<GoogleMap
mapContainerStyle={containerStyle}
center={center}
zoom={15}
onClick={mapClicked}
>
{markers.map((marker, index) => (
<Marker
key={index}
position={marker.position}
label={marker.label}
draggable={marker.draggable}
onDragEnd={event => markerDragEnd(event, index)}
onClick={event => markerClicked(marker, index)}
>
{
(activeInfoWindow === index)
&&
<InfoWindow
position={marker.position}
onCloseClick={() => setActiveInfoWindow(null)}
>
<b>{marker.position.lat}, {marker.position.lng}</b>
</InfoWindow>
}
</Marker>
))}
</GoogleMap>
);
};
export default MapComponent;
Note:- Don't forget to update your Google API Key.
You can easily add/remove markers. also, you can pass additional properties in the marker object based on your requirements.
(a). Add a marker
const allMarkers = [...markers];
const marker = {
position: {
lat: 28.625043,
lng: 79.810135
},
label: { color: "white", text: "P4" },
draggable: true
}
allMarkers.push(marker);
setMarkers(allMarkers)
(b). Remove a marker
Example:- Remove P4 marker
const filteredMarkers = markers.filter(marker => marker.label.text != "P4");
setMarkers(filteredMarkers)
(c). Update marker properties
Example:- Update P4 marker
const allMarkers = [...markers];
const index = allMarkers.findIndex(marker => marker.label.text == "P4");
if (index != -1) {
allMarkers[index]= { ...allMarkers[index], position: { lat: 28.625043, lng: 79.810135 } };
}
setMarkers(allMarkers)
5. Open the App.js file and make the following changes.
import React from "react";
import "./App.css";
import MapComponent from "./components/map.component";
function App() {
return (
<div className="App">
<h1>React Google Maps</h1>
<MapComponent/>
</div>
);
}
export default App;
6. Finally start the project.
npm start
open http://localhost:3000 in the browser.
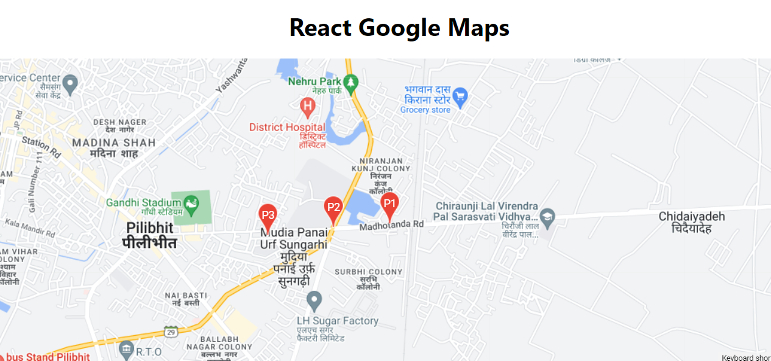
Extras:-
(a). Reverse Geocoding
const getAddress = (lat, lng) => {
const geocoder = new window.google.maps.Geocoder();
const latlng = new window.google.maps.LatLng(lat, lng);
const request = {
latLng: latlng
}
return new Promise((resolve, reject) => {
geocoder.geocode(request, results => {
results.length ? resolve(results[0].formatted_address) : reject(null);
});
})
}
You can use this function by passing lat and lng.
const mapClicked = async (event) => {
console.log(event.latLng.lat(), event.latLng.lng())
const address = await getAddress(event.latLng.lat(), event.latLng.lng())
console.log(address)
}
Checkout my full react-google-maps example.
https://github.com/ultimateakash/react-google-maps
If you facing any issues. don't hesitate to comment below. I will be happy to help you.
Thanks.
Leave Your Comment