How to Generate PDF in Laravel with DomPDF
Laravel-dompdf is an elegant wrapper around Dompdf. In this article, we gonna learn how to generate pdf in laravel.
1. Let's create a new laravel project.
composer create-project laravel/laravel laravel-pdf
2. After creating the project install the laravel-dompdf package.
composer require barryvdh/laravel-dompdf
3. Publish laravel dompdf config file.
php artisan vendor:publish --provider="Barryvdh\DomPDF\ServiceProvider"
This will create a dompdf.php file inside the config folder. this file contains all configurations of the laravel dompdf.
4. Create a Controller
php artisan make:controller PdfController
This will create PdfController.php inside app/Http/Controllers directory.
5. Open PdfController.php and add this code.
<?php
namespace App\Http\Controllers;
use Barryvdh\DomPDF\Facade\Pdf;
class PdfController extends Controller
{
public function print()
{
$invoiceItems = [
['item' => 'Website Design', 'amount' => 50.50],
['item' => 'Hosting (3 months)', 'amount' => 80.50],
['item' => 'Domain (1 year)', 'amount' => 10.50]
];
$invoiceData = [
'invoice_id' => 123,
'transaction_id' => 1234567,
'payment_method' => 'Paypal',
'creation_date' => date('M d, Y'),
'total_amount' => 141.50
];
$pdf = PDF::loadView('invoice', compact('invoiceItems', 'invoiceData'));
return $pdf->download('invoice.pdf');
}
}
Note:- In the above example we are using a view file(blade.php). we can also use raw HTML file.
PDF::loadFile(public_path().'/myfile.html');
or HTML string
$html = '<h1>Test</h1>'
PDF::loadHTML($html);
6. Create invoice.blade.php inside resources/views directory and add this code.
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Invoice Example</title>
<link rel="stylesheet" href="{{ asset('css/style.css') }}" />
</head>
<body>
<div class="invoice-box">
<table cellpadding="0" cellspacing="0">
<tr class="top">
<td colspan="2">
<table>
<tr>
<td class="title">
<img src="{{ asset('images/logo.png') }}" style="width: 100%; max-width: 88px" />
</td>
<td>
Invoice #: {{ $invoiceData['invoice_id'] }}<br />
Created: {{ $invoiceData['creation_date'] }}
</td>
</tr>
</table>
</td>
</tr>
<tr class="information">
<td colspan="2">
<table>
<tr>
<td>
Godaddy, LLC.<br />
12345 Sunny Road<br />
Sunnyville, CA 12345
</td>
<td>
Godaddy LLC.<br />
John Wick<br />
john@example.com
</td>
</tr>
</table>
</td>
</tr>
<tr class="heading">
<td>Payment Method</td>
<td># Transaction Id</td>
</tr>
<tr class="details">
<td>{{ $invoiceData['payment_method'] }}</td>
<td>{{ $invoiceData['transaction_id'] }}</td>
</tr>
<tr class="heading">
<td>Item</td>
<td>Amount</td>
</tr>
@foreach($invoiceItems as $invoiceItem)
<tr class="item @if($loop->last) last @endif">
<td>{{ $invoiceItem['item'] }}</td>
<td>${{ $invoiceItem['amount'] }}</td>
</tr>
@endforeach
<tr class="total">
<td></td>
<td>Total: ${{ $invoiceData['total_amount'] }}</td>
</tr>
</table>
</div>
</body>
</html>
7. Open routes/web.php and add the following code.
<?php
use App\Http\Controllers\PdfController;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/print', [PdfController::class, 'print']);
8. Finally open http://localhost/laravel-pdf/public/print in the browser.
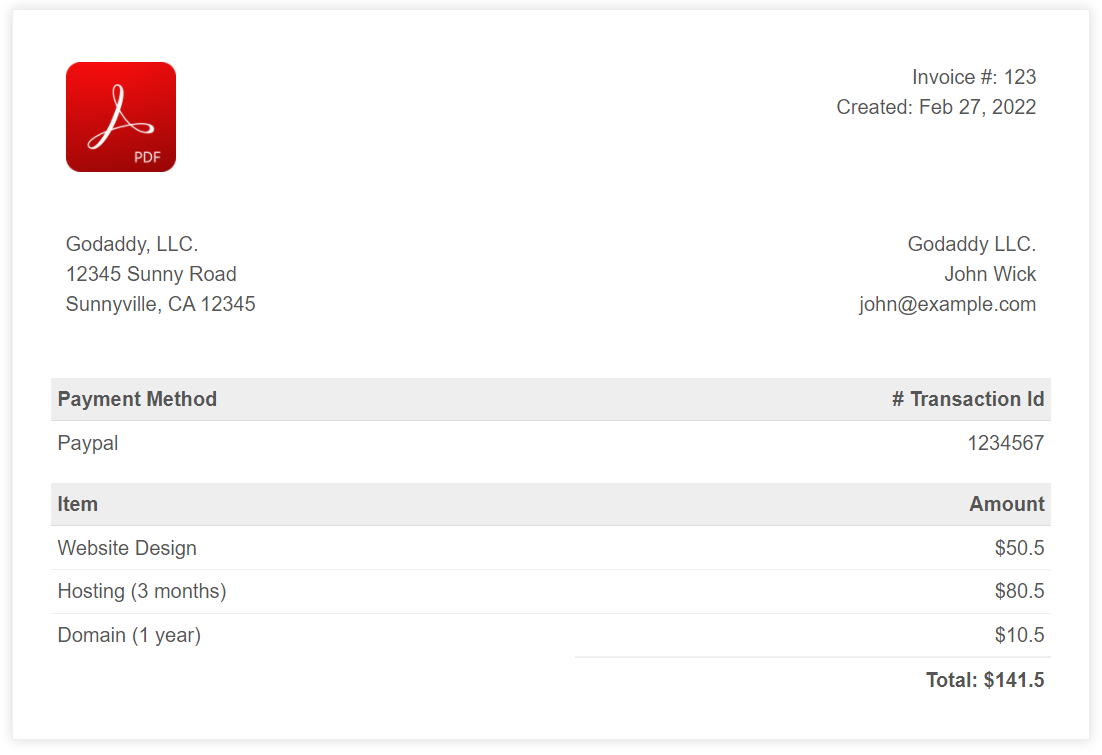
Extras:-
If you want to store the pdf then you can use save($filename) method.
PDF::loadView('invoice')->save(public_path('invoice.pdf'));
PDF::loadView('invoice')->save(storage_path('invoice.pdf'));
If you want to change the orientation or paper size then you can use setPaper($paper, $orientation)
PDF::loadView('invoice')
->setPaper('a4', 'landscape');
PDF::loadView('invoice')
->setPaper('letter', 'landscape')
->save(public_path('invoice.pdf'));
Checkout laravel-pdf repo. https://github.com/ultimateakash/laravel-pdf
If you facing any issues. don't hesitate to comment below. I will be happy to help you.
Thanks.
Leave Your Comment