How to Integrate Stripe Connect with Laravel 2022
Stripe Connect integrates quickly with any payment platform to easily accept money and payout third parties.
In this article, we will integrate stripe connect with our laravel project.
1. Let's create a new laravel project.
composer create-project laravel/laravel laravel-stripe-connect
2. Install stripe-php package.
composer require stripe/stripe-php
3. Create a stripe.php file inside the config folder and add this code.
<?php
return [
'api_keys' => [
'secret_key' => env('STRIPE_SECRET_KEY', null) // Secret KEY: https://dashboard.stripe.com/account/apikeys
],
'client_id' => env('STRIPE_CLIENT_ID', null), // Client ID: https://dashboard.stripe.com/account/applications/settings
'redirect_uri' => env('STRIPE_REDIRECT_URI', null), // Redirect Uri https://dashboard.stripe.com/account/applications/settings
'authorization_uri' => 'https://connect.stripe.com/oauth/authorize'
];
4. In your environment file(.env). create these environment variables.
STRIPE_CLIENT_ID=
STRIPE_SECRET_KEY=
STRIPE_REDIRECT_URI=http://localhost/laravel-stripe-connect/public/redirect
5. Now, we need to update these environment variables. let's go to stripe Settings and add your redirect url in the Redirects section. copy your client id and update it in your .env file.
Note:- For sandbox client id, click on test mode option on the stripe dashboard.
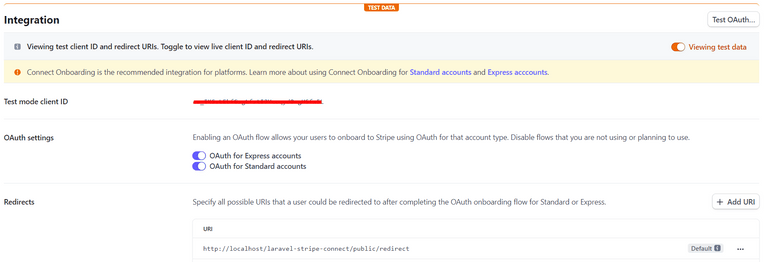
6. Now, in the stripe dashboard click on Developers. here click on API keys. Copy your secret key and update it in your .env file.
Note:- For sandbox keys, click on test mode option on the stripe dashboard.
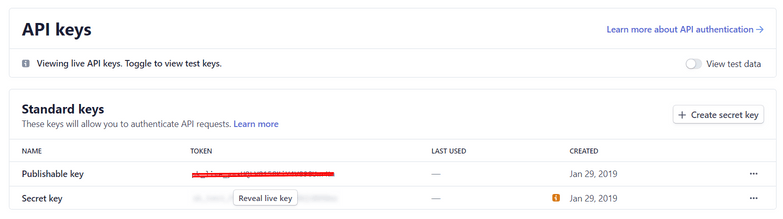
7. Next we need to create a button for authorization. create a file index.blade.php inside resources/views directory.
<div class='row'>
<div class='col d-flex align-items-center justify-content-center'>
<div class='card mt-5' style='width: 18rem;'>
<a href='{{ $connectUri }}'>
<img src="{{ asset('images/connect-purple.png') }}" class='img-thumbnail' alt='Connect With Stripe' />
</a>
</div>
</div>
</div>
Note:- Checkout github repo - https://github.com/ultimateakash/laravel-stripe-connect
8. Create a Controller
php artisan make:controller PaymentController
PaymentController.php
<?php
namespace App\Http\Controllers;
use Exception;
use Illuminate\Http\Request;
use Stripe\OAuth;
use Stripe\Stripe;
use Stripe\StripeClient;
class PaymentController extends Controller
{
private $stripe;
public function __construct()
{
$this->stripe = new StripeClient(config('stripe.api_keys.secret_key'));
Stripe::setApiKey(config('stripe.api_keys.secret_key'));
}
public function index()
{
$queryData = [
'response_type' => 'code',
'client_id' => config('stripe.client_id'),
'scope' => 'read_write',
'redirect_uri' => config('stripe.redirect_uri')
];
$connectUri = config('stripe.authorization_uri').'?'.http_build_query($queryData);
return view('index', compact('connectUri'));
}
public function redirect(Request $request)
{
$token = $this->getToken($request->code);
if(!empty($token['error'])) {
$request->session()->flash('danger', $token['error']);
return response()->redirectTo('/');
}
$connectedAccountId = $token->stripe_user_id;
$account = $this->getAccount($connectedAccountId);
if(!empty($account['error'])) {
$request->session()->flash('danger', $account['error']);
return response()->redirectTo('/');
}
return view('account', compact('account'));
}
private function getToken($code)
{
$token = null;
try {
$token = OAuth::token([
'grant_type' => 'authorization_code',
'code' => $code
]);
} catch (Exception $e) {
$token['error'] = $e->getMessage();
}
return $token;
}
private function getAccount($connectedAccountId)
{
$account = null;
try {
$account = $this->stripe->accounts->retrieve(
$connectedAccountId,
[]
);
} catch (Exception $e) {
$account['error'] = $e->getMessage();
}
return $account;
}
}
9. Open routes/web.php
<?php
use App\Http\Controllers\PaymentController;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', [PaymentController::class, 'index']);
Route::get('/redirect', [PaymentController::class, 'redirect']);
10. Finally open your project in the browser and click on the button.
http://localhost/laravel-stripe-connect/public/
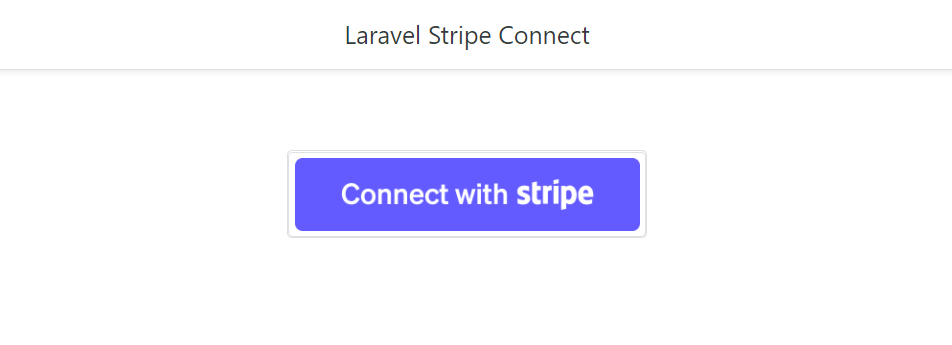
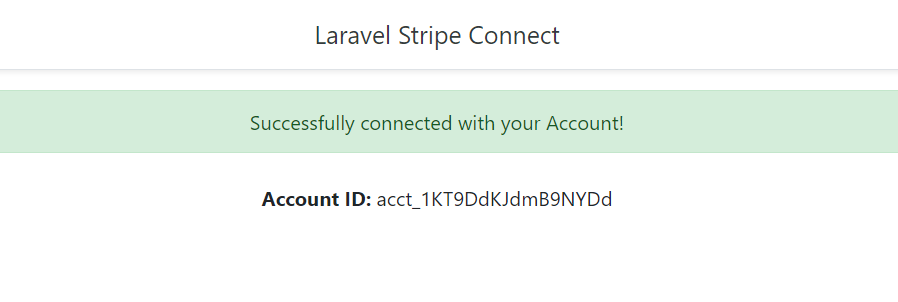
Checkout my full Stripe Connect example.
https://github.com/ultimateakash/laravel-stripe-connect
If you facing any issues. please post a comment. I will be happy to help you,
Thanks
Leave Your Comment