How To Implement Task Scheduling With Cron Job In Laravel 2022
Laravel's scheduler feature provides a convenient way to manage scheduled tasks for our web application. In this article, we will implement cronjob in laravel step by step.
1. Let's create a new laravel project.
composer create-project laravel/laravel laravel-cron
2. We need to create a console command. Laravel scheduler will execute these console commands based on the frequency.
php artisan make:command WeeklyNewsletter
This will create WeeklyNewsletter.php file inside app/Console/Commands directory.
3. Open WeeklyNewsletter.php and update command signature and description. also, update the handle() method.
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class WeeklyNewsletter extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'weekly:newsletter';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Send Weekly Newsletter to Users';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return int
*/
public function handle()
{
// Email Code Here
$this->log('Weekly newsletter sent successfully.');
}
}
4. Next we need to register our command. Open app/Console/Kernel.php. Here we need to call command() method by passing the console command signature also chained the frequency.
Note:- From Laravel v8.6.8 protected $commands property removed. so if you are using laravel version > v8.6.8 then you don't need to define $commands property.
Ref:- https://github.com/laravel/laravel/pull/5727
<?php
namespace App\Console;
use App\Console\Commands\WeeklyNewsletter;
use Illuminate\Console\Scheduling\Schedule;
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
class Kernel extends ConsoleKernel
{
protected $commands = [
WeeklyNewsletter::class
];
/**
* Define the application's command schedule.
*
* @param \Illuminate\Console\Scheduling\Schedule $schedule
* @return void
*/
protected function schedule(Schedule $schedule)
{
$schedule->command('weekly:newsletter')->weekly();
}
/**
* Register the commands for the application.
*
* @return void
*/
protected function commands()
{
$this->load(__DIR__.'/Commands');
require base_path('routes/console.php');
}
}
Here are some inbuilt frequency functions for task scheduling. Checkout all functions:- https://laravel.com/docs/8.x/scheduling
Method | Description |
---|---|
->cron('* * * * *'); |
Run the task on a custom cron schedule |
->everyMinute(); |
Run the task every minute |
->everyTwoMinutes(); |
Run the task every two minutes |
->everyThreeMinutes(); |
Run the task every three minutes |
->everyFourMinutes(); |
Run the task every four minutes |
->everyFiveMinutes(); |
Run the task every five minutes |
->everyTenMinutes(); |
Run the task every ten minutes |
->everyFifteenMinutes(); |
Run the task every fifteen minutes |
->everyThirtyMinutes(); |
Run the task every thirty minutes |
->hourly(); |
Run the task every hour |
->hourlyAt(17); |
Run the task every hour at 17 minutes past the hour |
->everyTwoHours(); |
Run the task every two hours |
->everyThreeHours(); |
Run the task every three hours |
->everyFourHours(); |
Run the task every four hours |
->everySixHours(); |
Run the task every six hours |
->daily(); |
Run the task every day at midnight |
->dailyAt('13:00'); |
Run the task every day at 13:00 |
->twiceDaily(1, 13); |
Run the task daily at 1:00 & 13:00 |
->weekly(); |
Run the task every Sunday at 00:00 |
->weeklyOn(1, '8:00'); |
Run the task every week on Monday at 8:00 |
->monthly(); |
Run the task on the first day of every month at 00:00 |
->monthlyOn(4, '15:00'); |
Run the task every month on the 4th at 15:00 |
->twiceMonthly(1, 16, '13:00'); |
Run the task monthly on the 1st and 16th at 13:00 |
->lastDayOfMonth('15:00'); |
Run the task on the last day of the month at 15:00 |
->quarterly(); |
Run the task on the first day of every quarter at 00:00 |
->yearly(); |
Run the task on the first day of every year at 00:00 |
->yearlyOn(6, 1, '17:00'); |
Run the task every year on June 1st at 17:00 |
->timezone('America/New_York'); |
Set the timezone for the task |
In case you are facing any problem with setting cron patterns you can checkout Crontab.guru - The cron schedule expression editor
Note:- You can also use the callback method if you don't want to create custom commands.
protected function schedule(Schedule $schedule)
{
$schedule->call(function () {
// Email Code Here
$this->info('Weekly newsletter sent successfully.');
})->weekly();
}
You can also use chaining.
protected function schedule(Schedule $schedule)
{
$schedule->call(function () {
// Email Code Here
$this->info('Weekly newsletter sent successfully.');
})->weekly()->sundays()->at('13:00');
}
5. If you want to test your console command on your local machine you can use three commands.
(a). Using signature
php artisan weekly:newsletter
(b). Using scheduler run
php artisan schedule:run
(c). Using scheduler worker
php artisan schedule:work
6. Finally we need to add our scheduler in the crontab of our server. so enter this command.
sudo crontab -e
and make changes like this.
# m h dom mon dow command
* * * * * php /path/to/artisan schedule:run >> /dev/null 2>&1
OR
* * * * * cd /path/to/project && php artisan schedule:run >> /dev/null 2>&1
In my case
# m h dom mon dow command
* * * * * cd /var/www/laravel-cron && php artisan schedule:run >> /dev/null 2>&1
You can check the crontab by hitting
sudo crontab -l
If you facing any issues. don't hesitate to comment below. I will be happy to help you.
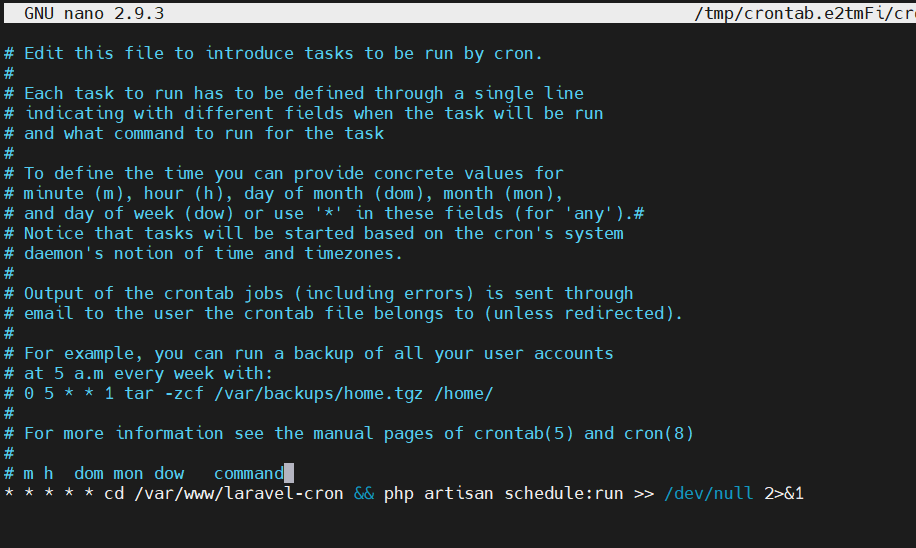
Leave Your Comment