How to Integrate Stripe Connect with Node.Js (Express) 2021
Stripe Connect integrates quickly with any payment platform to easily accept money and payout third parties.
In this article, we will integrate stripe connect with our node.js(express) project.
1. Let's create a new express project using express generator.
npm install -g express-generator
express node-stripe-connect --view=hbs //node-stripe-connect is project name
2. After creating the project. we need to install a few dependencies for stripe integration(stripe, http-build-query).
npm i stripe, http-build-query
3. create a new folder config inside your project. inside this folder create a file stripe.js
config/stripe.js
module.exports = {
clientId: process.env.STRIPE_CLIENT_ID, // Client ID: https://dashboard.stripe.com/account/applications/settings
secretKey: process.env.STRIPE_SECRET_KEY, // Secret KEY: https://dashboard.stripe.com/account/apikeys
redirectUri: process.env.STRIPE_REDIRECT_URI, // Redirect Uri https://dashboard.stripe.com/account/applications/settings
authorizationUri: 'https://connect.stripe.com/oauth/authorize'
}
4. Create a .env file and create these environment variables.
STRIPE_CLIENT_ID=
STRIPE_SECRET_KEY=
STRIPE_REDIRECT_URI=http://localhost:3000/redirect
5. Install dotenv npm package.
npm i dotenv
After Installation import dotenv in app.js
require('dotenv').config();
6. Now, we need to update these environment variables. let's go to stripe Settings and add your redirect url in the Redirects section. copy your client id and update it in your .env file.
Note:- For sandbox client id, click on test mode option on the stripe dashboard.
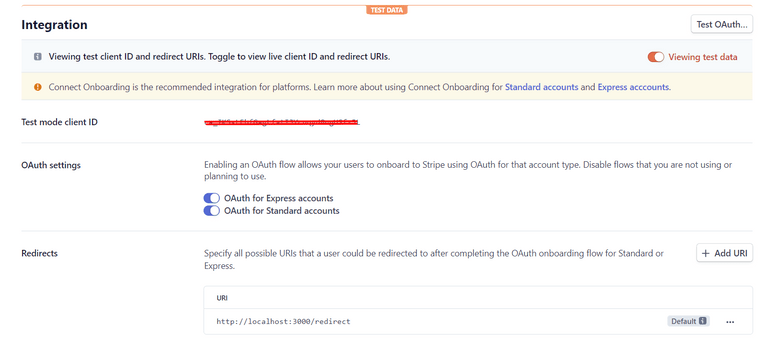
7. Now, in the stripe dashboard click on Developers. here click on API keys. Copy your secret key and update it in your .env file.
Note:- For sandbox keys, click on test mode option on the stripe dashboard.
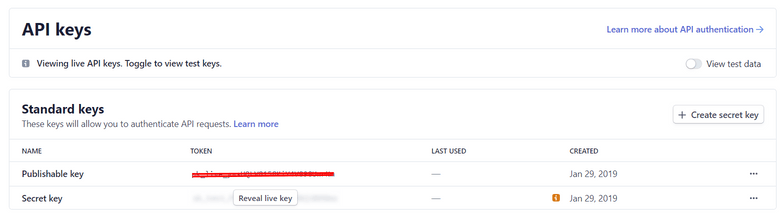
8. Next we need to create a button for authorization. Open views/index.hbs and add this code
<div class='row'>
<div class='col d-flex align-items-center justify-content-center'>
<div class='card mt-5' style='width: 18rem;'>
<a href='{{connectUri}}'>
<img src='/images/connect-purple.png' class='img-thumbnail' alt='Connect With Stripe' />
</a>
</div>
</div>
</div>
Note:- Checkout github repo - https://github.com/ultimateakash/node-stripe-connect
9. Create folder controllers and inside this create a file payment.controller.js and add this code.
const config = require('../config/stripe');
const stripe = require('stripe')(config.secretKey);
const httpBuildQuery = require('http-build-query');
const index = (req, res) => {
const queryData = {
response_type: 'code',
client_id: config.clientId,
scope: 'read_write',
redirect_uri: config.redirectUri
}
const connectUri = config.authorizationUri + '?' + httpBuildQuery(queryData);
res.render('index', { connectUri, error: req.flash('error') })
}
const redirect = async (req, res) => {
if (req.query.error) {
req.flash('error', req.query.error.error_description)
return res.redirect('/');
}
const token = await getToken(req.query.code);
if (token.error) {
req.flash('error', token.error)
return res.redirect('/');
}
const connectedAccountId = token.stripe_user_id;
const account = await getAccount(connectedAccountId);
if (account.error) {
req.flash('error', account.error)
return res.redirect('/');
}
res.render('account', { account });
}
const getToken = async (code) => {
let token = {};
try {
token = await stripe.oauth.token({ grant_type: 'authorization_code', code });
} catch (error) {
token.error = error.message;
}
return token;
}
const getAccount = async (connectedAccountId) => {
let account = {};
try {
account = await stripe.account.retrieve(connectedAccountId);
} catch (error) {
account.error = error.message;
}
return account;
}
module.exports = {
index,
redirect
}
10. Open routes/index.js and add this code
const express = require('express');
const router = express.Router();
const paymentController = require('../controllers/payment.controller');
router.get('/', paymentController.index)
router.get('/redirect', paymentController.redirect)
module.exports = router;
11. Finally start your project and open http://localhost:3000 and click on the button.
npm start
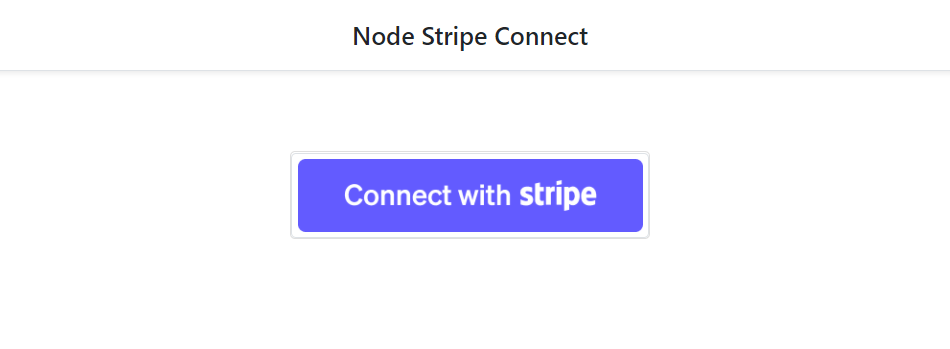
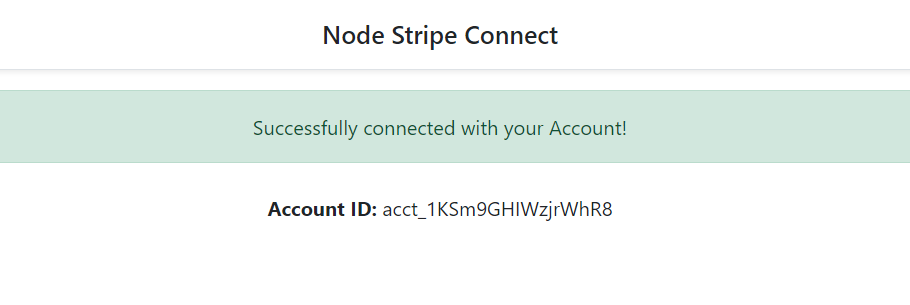
Checkout my full Stripe Connect example.
https://github.com/ultimateakash/node-stripe-connect
If you facing any issues. please post a comment. I will be happy to help you,
Thanks
Leave Your Comment