How To Integrate Leaflet Maps in Laravel 2022
Leaflet is an open-source JavaScript library for mobile-friendly interactive maps. In this tutorial, we will integrate leaflet maps in our laravel project.
1. Let's create a new laravel project.
composer create-project laravel/laravel laravel-leaflet-maps
2. Create a view and add the following code.
<!DOCTYPE html>
<html>
<head>
<meta charset='utf-8'>
<meta name='viewport' content='width=device-width, initial-scale=1'>
<style>
.text-center {
text-align: center;
}
#map {
width: '100%';
height: 400px;
}
</style>
<link rel='stylesheet' href='https://unpkg.com/leaflet@1.8.0/dist/leaflet.css' crossorigin='' />
</head>
<body>
<h1 class='text-center'>Laravel Leaflet Maps</h1>
<div id='map'></div>
<script src='https://unpkg.com/leaflet@1.8.0/dist/leaflet.js' crossorigin=''></script>
<script>
let map, markers = [];
/* ----------------------------- Initialize Map ----------------------------- */
function initMap() {
map = L.map('map', {
center: {
lat: 28.626137,
lng: 79.821603,
},
zoom: 15
});
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: '© OpenStreetMap'
}).addTo(map);
map.on('click', mapClicked);
initMarkers();
}
initMap();
/* --------------------------- Initialize Markers --------------------------- */
function initMarkers() {
const initialMarkers = <?php echo json_encode($initialMarkers); ?>;
for (let index = 0; index < initialMarkers.length; index++) {
const data = initialMarkers[index];
const marker = generateMarker(data, index);
marker.addTo(map).bindPopup(`<b>${data.position.lat}, ${data.position.lng}</b>`);
map.panTo(data.position);
markers.push(marker)
}
}
function generateMarker(data, index) {
return L.marker(data.position, {
draggable: data.draggable
})
.on('click', (event) => markerClicked(event, index))
.on('dragend', (event) => markerDragEnd(event, index));
}
/* ------------------------- Handle Map Click Event ------------------------- */
function mapClicked($event) {
console.log(map);
console.log($event.latlng.lat, $event.latlng.lng);
}
/* ------------------------ Handle Marker Click Event ----------------------- */
function markerClicked($event, index) {
console.log(map);
console.log($event.latlng.lat, $event.latlng.lng);
}
/* ----------------------- Handle Marker DragEnd Event ---------------------- */
function markerDragEnd($event, index) {
console.log(map);
console.log($event.target.getLatLng());
}
</script>
</body>
</html>
#Add a marker
const data = {
position: { lat: 28.625043, lng: 79.810135 },
draggable: true
}
const marker = generateMarker(data, markers.length - 1);
marker.addTo(map).bindPopup(`<b>${data.position.lat}, ${data.position.lng}</b>`);
markers.push(marker);
}
#Remove a marker
map.removeLayer(markers[index])
markers.splice(index, 1)
#Update a marker
markers[index].setLatLng({ lat: 28.625043, lng: 79.810135 });
setOptions(options) - set marker properties
setPosition(latlng) - set marker position
setLabel(label) - set marker label
3. Create a controller and add the following code.
php artisan make:controller MapController
app/Http/Controllers/MapController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class MapController extends Controller
{
public function index()
{
$initialMarkers = [
[
'position' => [
'lat' => 28.625485,
'lng' => 79.821091
],
'draggable' => true
],
[
'position' => [
'lat' => 28.625293,
'lng' => 79.817926
],
'draggable' => false
],
[
'position' => [
'lat' => 28.625182,
'lng' => 79.81464
],
'draggable' => true
]
];
return view('map', compact('initialMarkers'));
}
}
4. Create Route
routes/web.php
<?php
use App\Http\Controllers\MapController;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', [MapController::class, 'index']);
5. Finally open http://localhost/laravel-leaflet-maps/public in the browser.
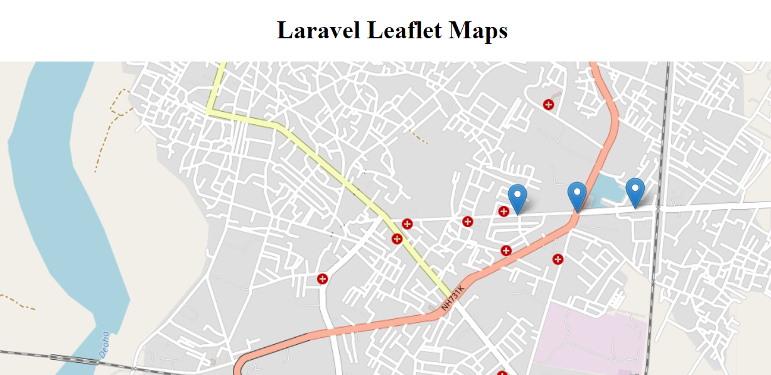
Extras:- Reverse Geocoding
Add leaflet-control-geocoder script.
<script src='https://unpkg.com/leaflet-control-geocoder@2.4.0/dist/Control.Geocoder.js'></script>
const geocoder = L.Control.Geocoder.nominatim();
geocoder.reverse(
{ lat: 28.625043, lng: 79.810135 },
map.getZoom(),
(results) => {
if(results.length) {
console.log("formatted_address", results[0].name)
}
}
);
Checkout laravel-leaflet-maps repo. https://github.com/ultimateakash/laravel-leaflet-maps
Leave Your Comment