How to Send Email in Node.js 2022
Nodemailer provides a convenient way to send emails. In this article, we gonna learn how to send emails in node.js.
1. Let's create a new express project using express generator.
npm i -g express-generator
express node-mail --view=hbs
cd node-mail
2. Create a folder config and inside this create a file mail.js.
config/mail.js
module.exports = {
host: process.env.MAIL_HOST,
port: process.env.MAIL_PORT,
username: process.env.MAIL_USERNAME,
password: process.env.MAIL_PASSWORD,
from_address: process.env.MAIL_FROM_ADDRESS,
from_name: process.env.MAIL_FROM_NAME
}
3. Install dotenv npm package.
npm i dotenv
After Installation import dotenv in app.js
require('dotenv').config();
4. Create a .env file in the root and add these environment variables.
MAIL_HOST=
MAIL_PORT=
MAIL_USERNAME=
MAIL_PASSWORD=
MAIL_FROM_ADDRESS=
MAIL_FROM_NAME=
Note:- Update your email credentials.
5. Install nodemailer npm package.
npm i nodemailer
6. Create a utils folder. Inside this folder create mailer.js
utils/mailer.js
const nodemailer = require('nodemailer');
const config = require('../config/mail');
const transporter = nodemailer.createTransport({
host: config.host,
port: config.port,
secure: false, // true for 465, false for other ports
auth: {
user: config.username,
pass: config.password
},
},{
from: `${config.from_name} <${config.from_address}>`
});
exports.sendMail = (fields) => transporter.sendMail(fields);
Note:- createTransport() takes two arguments
createTransport(transport, defaults)
You can override default properties by passing them into sendMail() function.
7. Create a folder controllers and inside this create notification.controller.js
controllers/notification.controller.js
const { sendMail } = require('../utils/mailer');
const hbs = require('hbs');
const fs = require('fs');
const readFile = require('util').promisify(fs.readFile);
exports.example1 = async (req, res) => {
const email = 'akashmjp@gmail.com';
const article = {
title: 'Lorem Ipsum',
description: 'Lorem Ipsum is simply dummy text of the printing and typesetting industry.'
}
const content = await readFile('views/emails/newsletter.hbs','utf8');
const template = hbs.compile(content);
const html = template({ article });
await sendMail({
to: email,
subject: 'Weekly Newsletter',
html: html
})
return res.send('Email Sent. - Example1')
}
exports.example2 = async (req, res) => {
const email = 'akashmjp@gmail.com';
const text = 'Lorem Ipsum is simply dummy text of the printing and typesetting industry.'
await sendMail({
to: email,
subject: 'Weekly Newsletter',
text: text
})
return res.send('Email Sent. - Example2')
}
Here is a list of the useful properties of fields object:
from?: string | Address | undefined;
sender?: string | Address | undefined;
to?: string | Address | Array<string | Address> | undefined;
cc?: string | Address | Array<string | Address> | undefined;
bcc?: string | Address | Array<string | Address> | undefined;
replyTo?: string | Address | undefined;
subject?: string | undefined;
text?: string | Buffer | Readable | AttachmentLike | undefined;
html?: string | Buffer | Readable | AttachmentLike | undefined;
headers?: Headers | undefined;
attachments?: Attachment[] | undefined;
priority?: "high"|"normal"|"low" | undefined;
You can also pass to property as an array of emails.
const emails = ['user1@gmail.com', 'user1@gmail.com'];
const text = 'Lorem Ipsum is simply dummy text of the printing and typesetting industry.'
await sendMail({
to: emails,
subject: 'Weekly Newsletter',
text: text
})
Email with Attachment
await sendMail({
to: email,
subject: 'Weekly Newsletter',
text: text,
attachments: [
{
path: 'https://www.ultimateakash.com/assets/img/logo/logo.png'
}
]
})
you can also pass a custom name for the attachment.
{
filename: 'mylogo.png',
path: 'https://www.ultimateakash.com/assets/img/logo/logo.png'
}
Ref:- Checkout https://nodemailer.com/message/attachments
8. Update routes.
routes/index.js
const express = require('express');
const router = express.Router();
const notificationController = require('../controllers/notification.controller');
router.get('/example1', notificationController.example1);
router.get('/example2', notificationController.example2);
module.exports = router;
9. Finally, start the project and open http://localhost:3000/example1
npm start
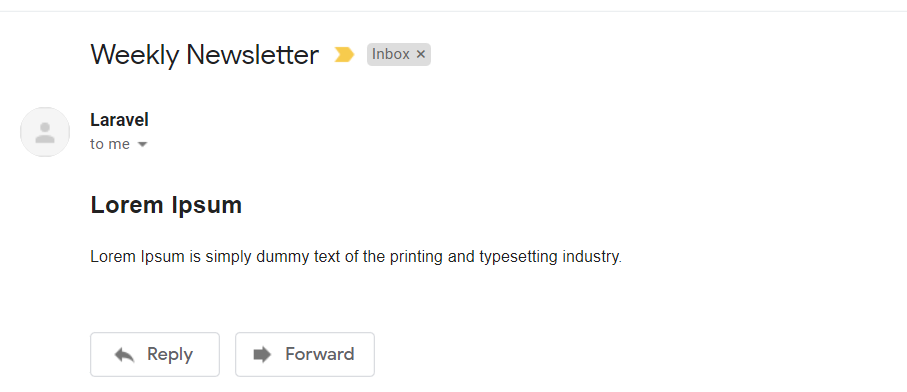
Github Repo: https://github.com/ultimateakash/node-mail
Leave Your Comment