How To Integrate Google Analytics in Angular 2022
Google Analytics is a powerful tool for tracking and analyzing online traffic. In this article, we gonna learn how to integrate google analytics in angular application step by step.
1. Go to https://analytics.google.com
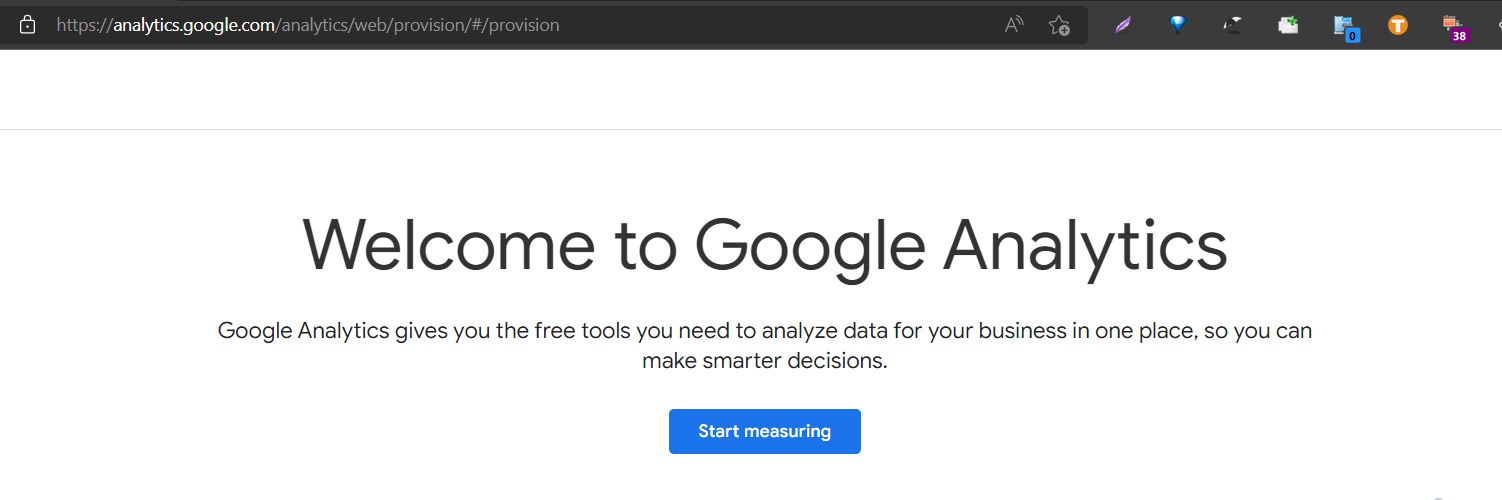
2. Create an account and property.
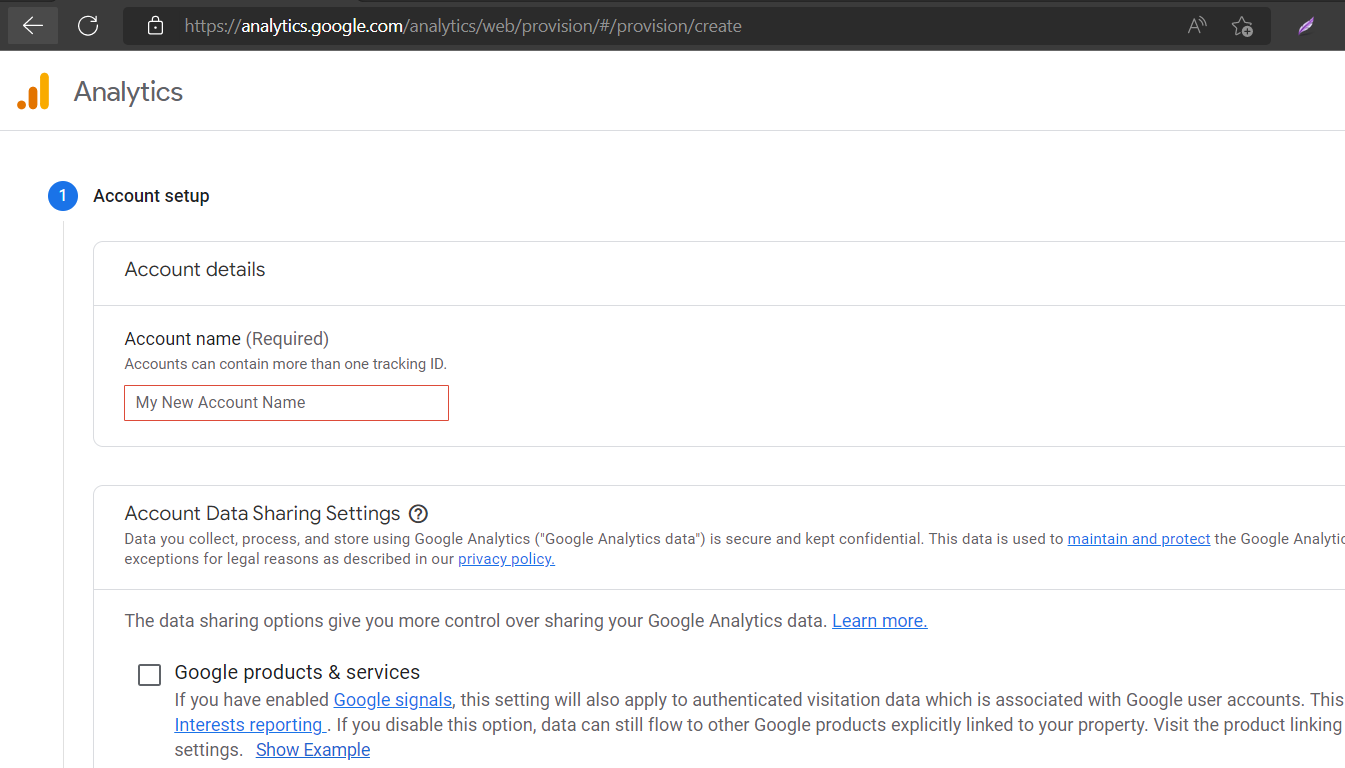
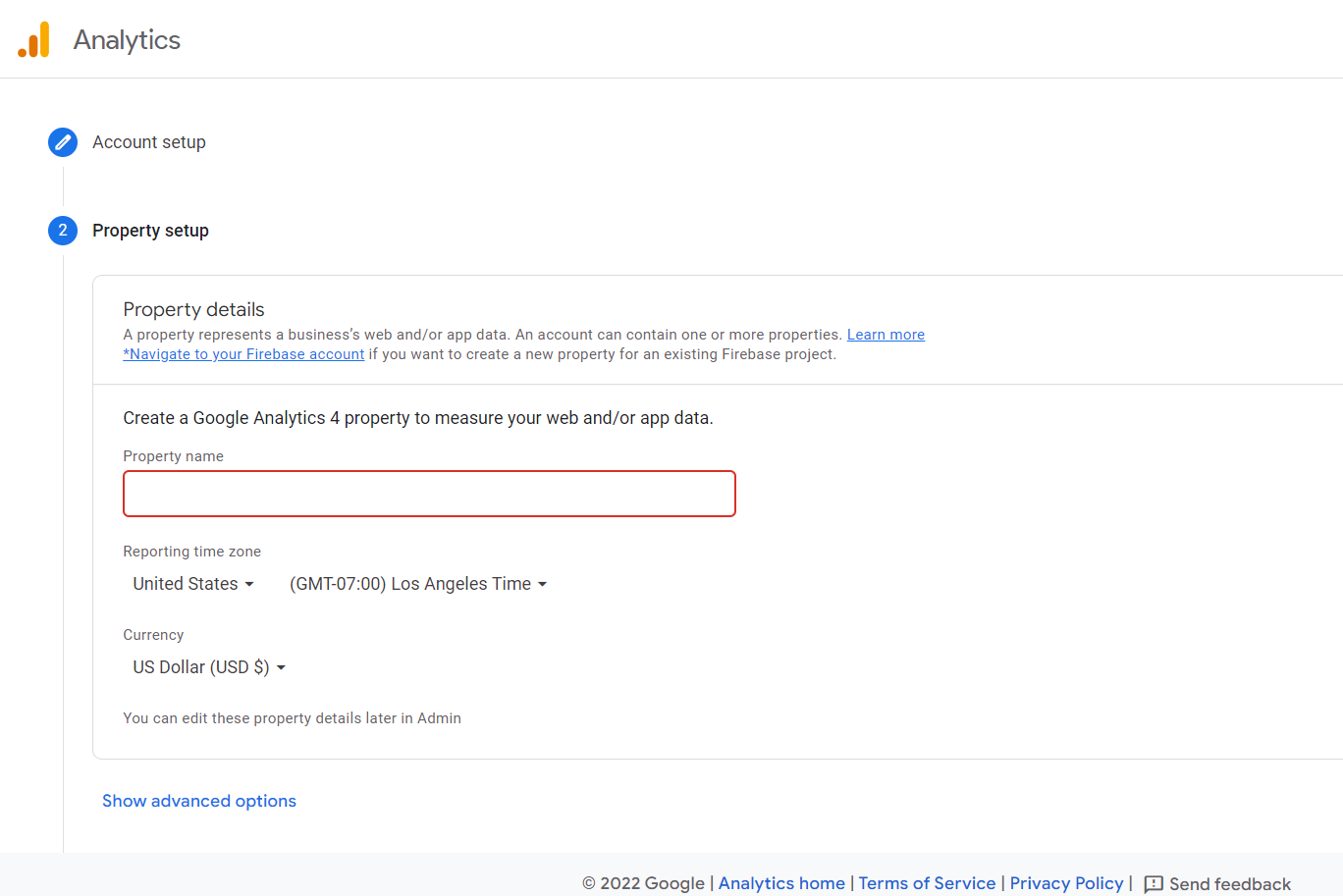
3. Create a data stream.
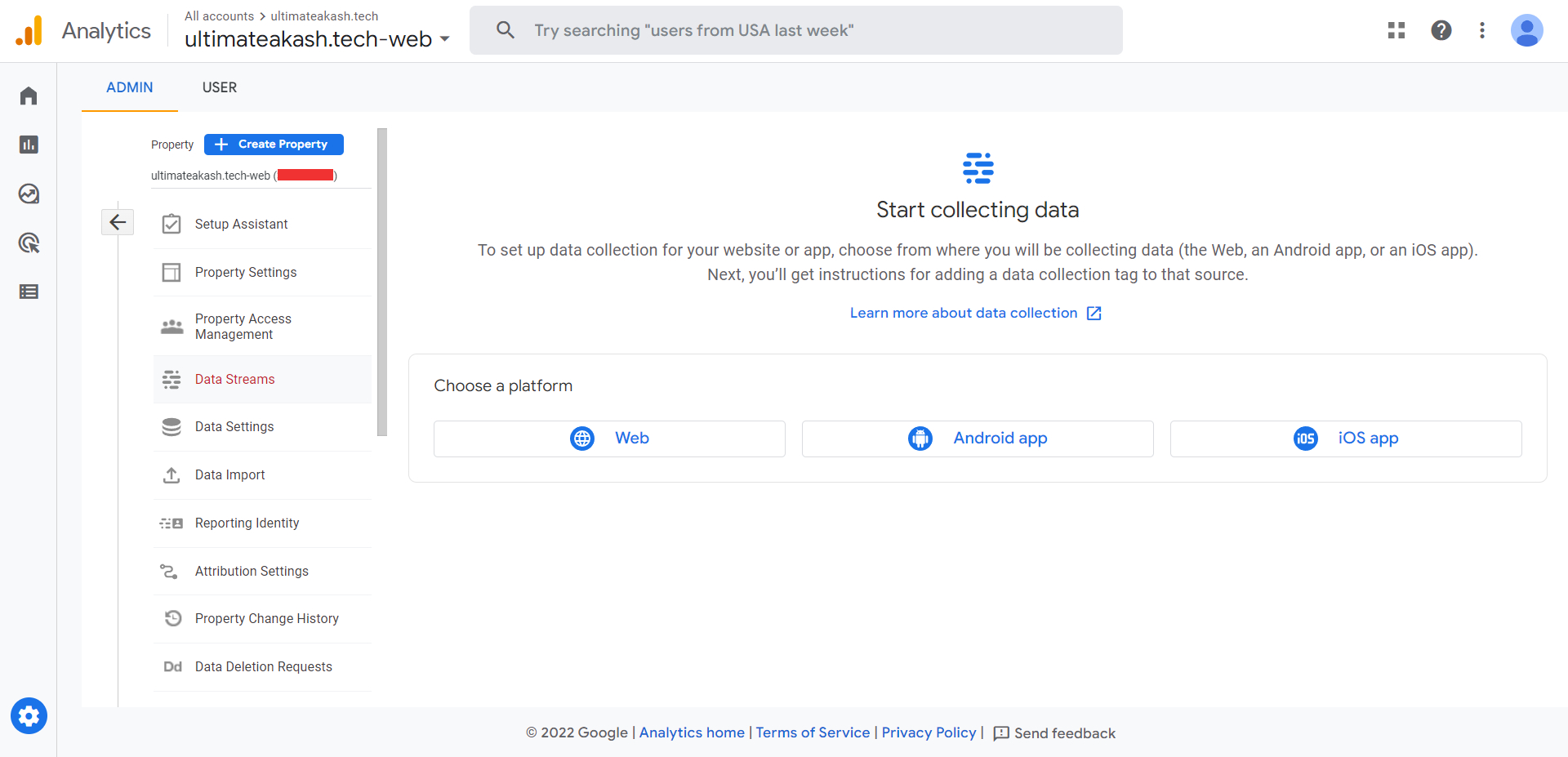
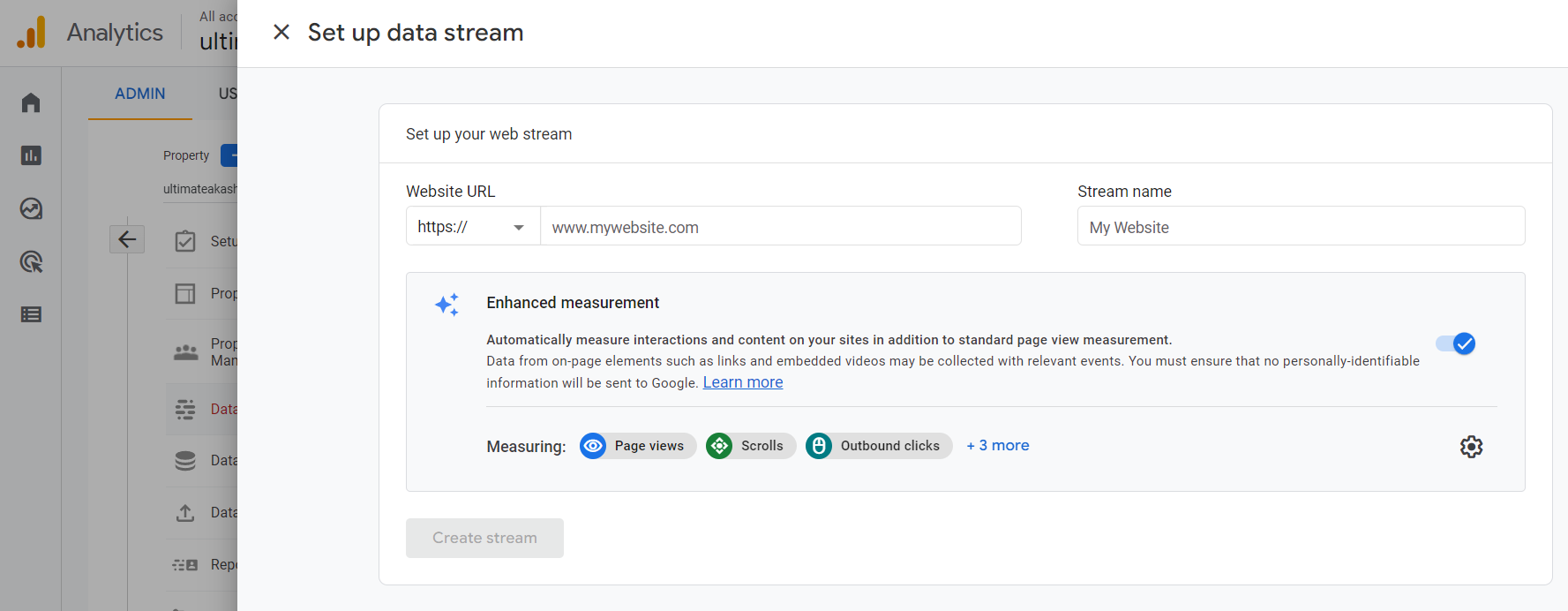
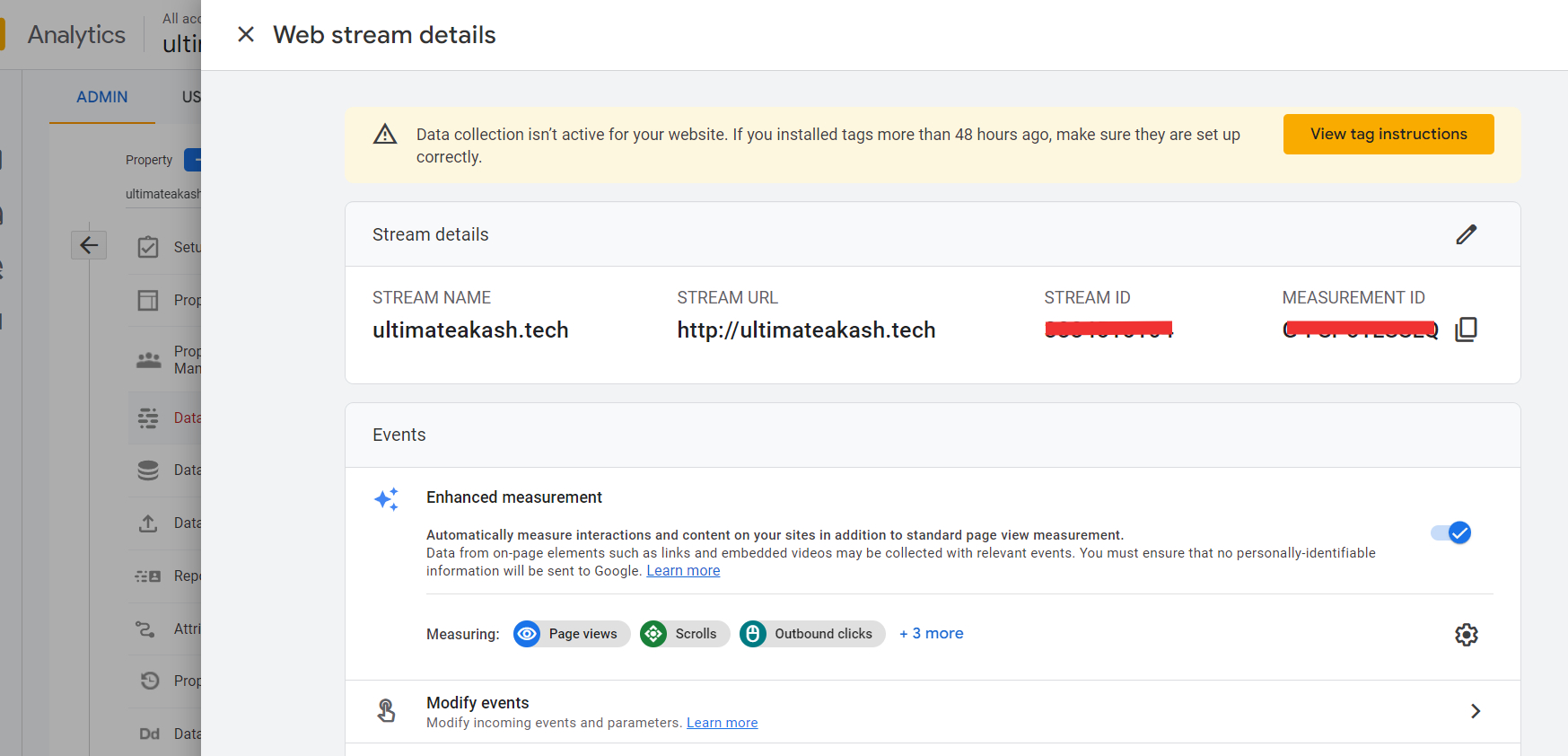
4. Click on view tag instructions
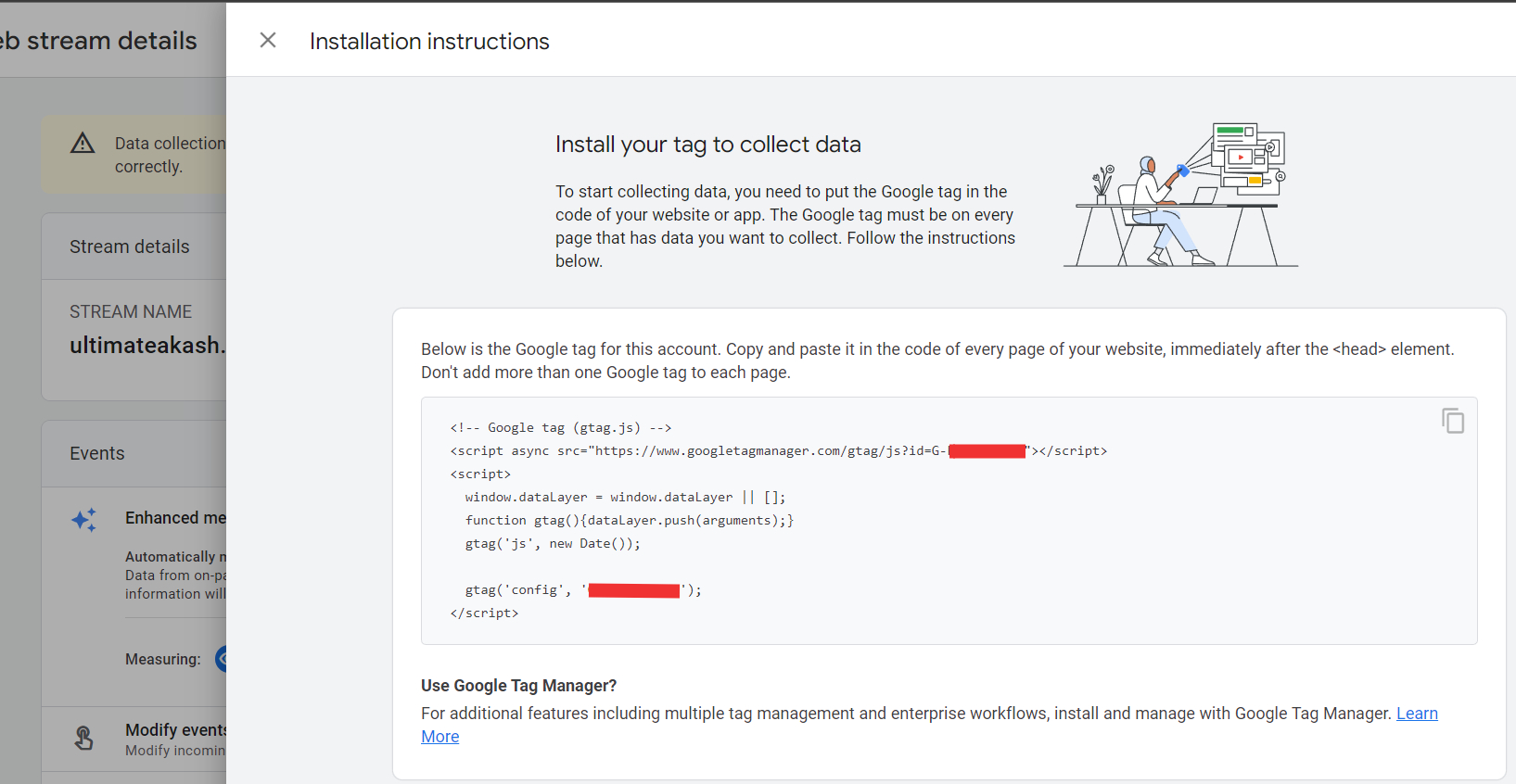
next, we need to add the above code in our angular application.
5. Let's install @angular/cli package.
npm i -g @angular/cli
6. Create a new angular project.
ng new angular-google-analytics --routing
cd angular-google-analytics
7. Install @types/gtag.js npm package.
npm install @types/gtag.js --save-dev
8. Open tsconfig.app.json and update types property.
/* To learn more about this file see: https://angular.io/config/tsconfig. */
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/app",
"types": ["gtag.js"]
},
"files": [
"src/main.ts",
"src/polyfills.ts"
],
"include": [
"src/**/*.d.ts"
]
}
9. Open index.html and add google tag code(step 4)
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Demo App</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
<!-- Google tag (gtag.js) -->
<script async src="https://www.googletagmanager.com/gtag/js?id=G-XXXXXXXXXX"></script>
<script>
window.dataLayer = window.dataLayer || [];
function gtag() { dataLayer.push(arguments); }
gtag('js', new Date());
gtag('config', 'G-XXXXXXXXXX');
</script>
</head>
<body>
<app-root></app-root>
</body>
</html>
10. Create a few components
ng g c home --skipTests=true
ng g c aboutUs --skipTests=true
ng g c contactUs --skipTests=true
ng g c notFound --skipTests=true
11. Open app-routing.module.ts and update routes.
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { HomeComponent } from './home/home.component';
import { AboutUsComponent } from './about-us/about-us.component';
import { ContactUsComponent } from './contact-us/contact-us.component';
import { NotFoundComponent } from './not-found/not-found.component';
const routes: Routes = [
{
path: '',
component: HomeComponent,
pathMatch: 'full',
data: { title: 'Homepage' }
},
{
path: 'about-us',
component: AboutUsComponent,
data: { title: 'About Us' }
},
{
path: 'contact-us',
component: ContactUsComponent,
data: { title: 'Contact Us' }
},
{
path: '**',
component: NotFoundComponent,
data: { title: 'Not Found' }
}
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
12. Open app.component.html and add the following code.
<ul>
<li><a routerLink='/'>Home</a></li>
<li><a routerLink='/about-us'>About Us</a></li>
<li><a routerLink='/contact-us'>Contact Us</a></li>
</ul>
<router-outlet></router-outlet>
13. Open app.component.ts and add the following code.
import { DOCUMENT } from '@angular/common';
import { Component, Inject } from '@angular/core';
import { Title } from '@angular/platform-browser';
import { ActivatedRoute, NavigationEnd, Router, RouterState } from '@angular/router';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(
private router: Router,
private titleService: Title,
@Inject(DOCUMENT) private document: Document
) {
this.handleRouteEvents();
}
handleRouteEvents() {
this.router.events.subscribe(event => {
if (event instanceof NavigationEnd) {
const title = this.getTitle(this.router.routerState, this.router.routerState.root).join('-');
this.titleService.setTitle(title);
gtag('event', 'page_view', {
page_title: title,
page_path: event.urlAfterRedirects,
page_location: this.document.location.href
})
}
});
}
getTitle(state: RouterState, parent: ActivatedRoute): string[] {
const data = [];
if (parent && parent.snapshot.data && parent.snapshot.data['title']) {
data.push(parent.snapshot.data['title']);
}
if (state && parent && parent.firstChild) {
data.push(...this.getTitle(state, parent.firstChild));
}
return data;
}
}
gtag.js event command
gtag('event', eventName, eventParameters);
events ref:- https://developers.google.com/analytics/devguides/collection/gtagjs/events
page_view event ref:- https://developers.google.com/analytics/devguides/collection/gtagjs/pages
14. Finally start the project and open http://localhost:4200
npm start
Open analytics reports.
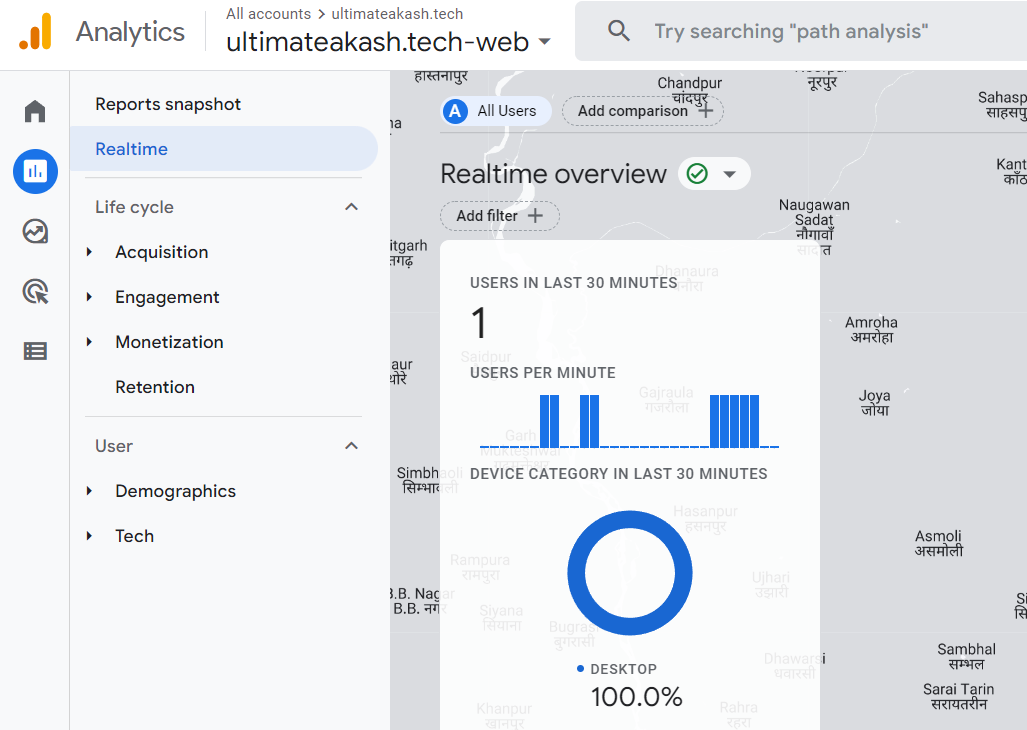
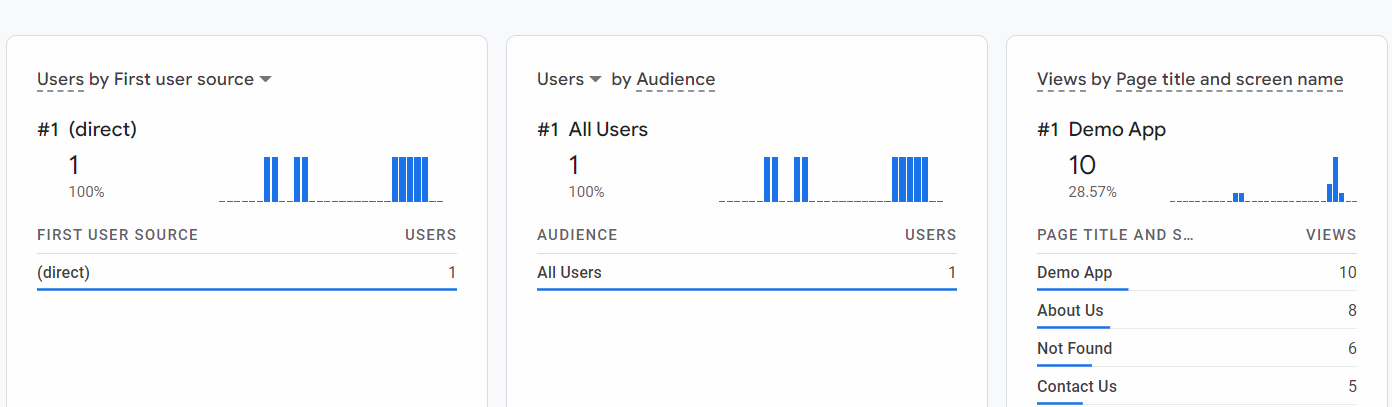
Checkout my full angular-google-analytics example.
https://github.com/ultimateakash/angular-google-analytics
If you facing any issues. don't hesitate to comment below.
Thanks.
Angular Universal (SSR) Solution - Cannot find name 'gtag' · Issue #1 · ultimateakash/angular-google-analytics (github.com)
Leave Your Comment